A multi-dimensional array can be termed as an array of arrays that stores homogeneous data in tabular form. Data in multidimensional arrays are stored in row-major order.
The general form of declaring N-dimensional arrays is:
data_type array_name[size1][size2]....[sizeN];
data_type: Type of data to be stored in the array.
array_name: Name of the array
size1, size2,… ,sizeN: Sizes of the dimension
Examples:
Two dimensional array: int two_d[10][20];
Three dimensional array: int three_d[10][20][30];
Size of Multidimensional Arrays:
The total number of elements that can be stored in a multidimensional array can be calculated by multiplying the size of all the dimensions.
For example:
The array int x[10][20] can store total (10*20) = 200 elements.
Similarly array int x[5][10][20] can store total (5*10*20) = 1000 elements.
IV.4. Jagged Array
Jagged array is a array of arrays such that member arrays can be of different sizes. In other words, the length of each array index can differ. The elements of Jagged Array are reference types and initialized to null by default. Jagged Array can also be mixed with multidimensional arrays. Here, the number of rows will be fixed at the declaration time, but you can vary the number of columns.
Declaration
In Jagged arrays, user has to provide the number of rows only. If the user is also going to provide the number of columns, then this array will be no more Jagged Array.
Syntax:
data_type[][] name_of_array = new data_type[rows][]
Example:
int[][] jagged_arr = new int[4][]
In the above example, a single-dimensional array is declared that has 4 elements(rows), each of which is a 1-D array of integers.
Initialization
The elements of Jagged Array must be initialized before its use. You can separately initialize each array element. There are many ways to initialize the Jagged array’s element.
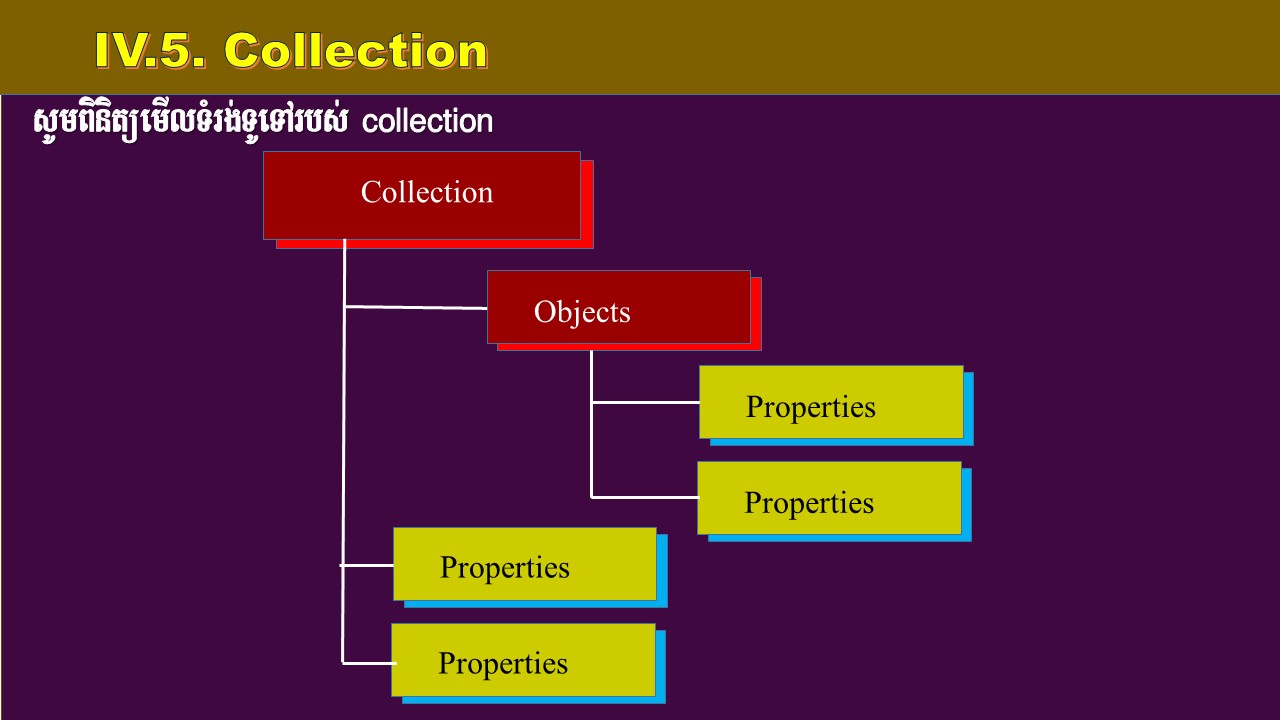
0 Comments